Codeigniter4 provides a pagination library that is simple to use. Let us consider an example. Below is a table structure in mysql .
CREATE TABLE `Persons` (
`id` int NOT NULL AUTO_INCREMENT,
`firstname` varchar(50) DEFAULT NULL,
`lastname` varchar(50) DEFAULT NULL,
`age` int DEFAULT NULL,
`address` varchar(255) DEFAULT NULL,
`pincode` int DEFAULT NULL,
`created_date` datetime DEFAULT NULL,
`updated_date` datetime DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4
INSERT INTO `Persons` (`firstname`,`lastname`,`age`,`address`,`pincode`)
VALUES
("Quamar","Avila","23","Bình Phước, Germany","773499"),
("Yoko","Clarke","40","Bellstown South Africa","361356"),
("Ron","Weasley","24","Burrow,Scotland","678432"),
("Peter","Parker","42","Spiderverse","371296"),
("Alice","Cullen","19","Forks","442649");
Now lets see the controller first in app/Controllers/Home.php
<?php
namespace App\Controllers;
use App\Models;
class Home extends BaseController
{
public function index()
{
$pager = \Config\Services::pager();
$request = \Config\Services::request();
$userModel = new \App\Models\UserModel();
$page = (int) ($request->getGet('page') ?? 1);
$limit = $userModel->limit;
$offset = ($page-1)*$limit;
$allPersons = $userModel->findAll($limit, $offset);
$allPersonsCount = $userModel->countAll();
$data["users"] = $allPersons;
$data['pager'] = $pager;
$data['page_links'] = $pager->makelinks($page,$userModel->limit,$allPersonsCount);
return view('users/home',$data);
}
}
We created the pagination object with the line.
$pager = \Config\Services::pager();
The links for each page are created by default with query parameter ie. page=1 or page=2 and so on.
The $pager->makelinks method takes three parameters in this case.
1. page number
2. Number of records per page.
3. Total number of records.
Here we will cover only 3 parameters. There are more parameters, which we have shown in case of custom pagination design in another post.
In the model file we will set the limit variable as 2, to show two records on a page.
When we pass these the page links are created automatically.
Lets test this with the model and view pages.
Model is created at app/Models/UserModel.php as below.
<?php
namespace App\Models;
use CodeIgniter\Model;
class UserModel extends Model{
protected $table = 'Persons';
protected $primaryKey = 'id';
protected $useAutoIncrement = true; //whether the mentioned primaryKey is an autoincrement field
protected $dateFormat = 'datetime';
protected $createdField = 'created_date';
protected $updatedField = 'updated_date';
protected $useTimestamps = true;
protected $allowedFields = ["firstname","lastname","age","address","pincode"];
protected $limit = 2;
public function __construct(){
parent::__construct();
$this->db = \Config\Database::connect();
}
public function getAllUsers(){
$builder = $this->db->table($this->table." p ");
$builder->select('p.firstname, p.lastname, p.age');
$query = $builder->get();
$users = $query->getResultArray(); //returns response as array
return $users;
}
}
?>
The view file is created at app/Views/users/home.php
<style>
ul {
list-style-type: none;
margin: 0;
padding: 0;
overflow: hidden;
}
li {
float: left;
}
li a {
display: block;
text-align: center;
padding: 16px;
}
</style>
<table border="1">
<tr>
<th>Firstname</th>
<th>Lastname</th>
</tr>
<?php
foreach($users as $u) {
$row = "<tr>";
$row .= "<td>".$u["firstname"]."</td>";
$row .= "<td>".$u["lastname"]."</td>";
$row .= "</tr>";
echo $row;
}
?>
</table>
<?php if($page_links){
echo $page_links;
} ?>
When you run this on the localhost, we will see that 3 pagination links are created with 2 records on each page as we have set the limit. Below is a screenshot of the output. We can see the page number in the url along with pagination links.
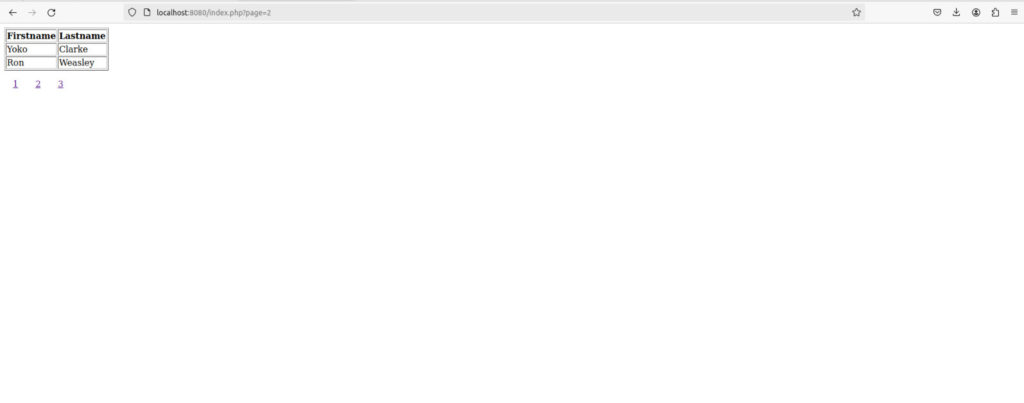