Codeigniter4 provides an easy data validation class to validate different types of inputs.
In the below example, we will use a form with username, email and password fields and validate them with required and valid_email options.
On submit of the form, the controller will receive the inputs and run the validation rules.
- Create the controller Form.php under App\Controller folder.
<?php
namespace App\Controllers;
use CodeIgniter\Controller;
class Form extends Controller
{
function __construct()
{
$this->validation = \Config\Services::validation();
$this->input = \Config\Services::request();
}
public function index(){
$data = array();
$data['validation'] = $this->validation; //Capture validation errors
$data['input'] = $this->input->getPost();
if($this->input->getPost('register')) {
//set validation Rules
$this->validation->setRule('username', 'User Name', 'required');
$this->validation->setRule('email', 'Email', 'required|valid_email');
$this->validation->setRule('password', 'Password', 'required');
//Run validation rule
if($this->validation->run($this->input->getPost()) ){
echo "Validation successful";
print_r($this->input->getPost());
exit;
//Proceed to process the record and redirect
}
}
echo view("client",$data);
}
}
2. Create the view file client.php under App\Views folder
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.4/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script>
<div class="container">
<div class="content">
<form class="form-horizontal" action='' method="POST">
<fieldset>
<div id="legend">
<legend class="">Register</legend>
</div>
<div class="control-group">
<label class="control-label" for="username">Username</label>
<div class="controls">
<input type="text" id="username" name="username" value="<?=isset($input['username']) ? $input['username'] : ""?>" placeholder="" class="input-xlarge">
<p class="alert-danger"><?php echo $validation->getError('username');?></p>
</div>
</div>
<div class="control-group">
<label class="control-label" for="email">E-mail</label>
<div class="controls">
<input type="text" id="email" name="email" value="<?=isset($input['email']) ? $input['email'] : ""?>" placeholder="" class="input-xlarge">
<p class="alert-danger"><?php echo $validation->getError('email');?></p>
</div>
</div>
<div class="control-group">
<label class="control-label" for="password">Password</label>
<div class="controls">
<input type="password" id="password" value="<?=isset($input['password']) ? $input['password'] : ""?>" name="password" placeholder="" class="input-xlarge">
<p class="alert-danger"><?php echo $validation->getError('password');?></p>
</div>
</div>
<div class="control-group">
<div class="controls">
<button class="btn btn-success" value="register" name="register">Register</button>
</div>
</div>
</fieldset>
</form>
</div>
</div>
3. Add routes to view the page in browser.
$routes->get('validation/form', 'Controllers\Form::index');
$routes->post('validation/form', 'Controllers\Form::index');
4. When you view the page at http://localhost:8080/validation/form on your local setup, you will see the page as below.
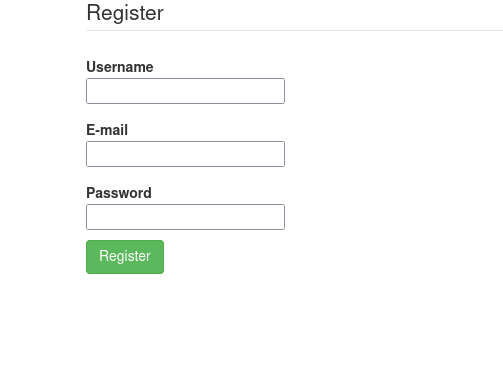
5. When you click the register button, the inputs are validated at the Controller Form.php and can be seen as below.
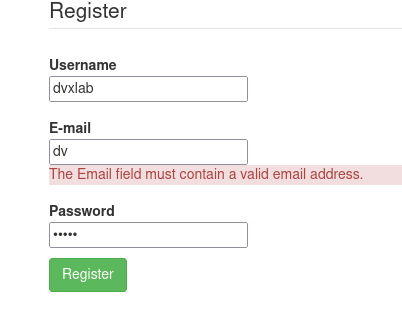